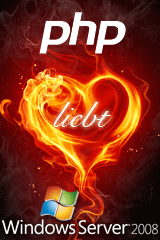
:: Anbieterverzeichnis ::
Globale Branchen
Informieren Sie sich über ausgewählte
Unternehmen im
Anbieterverzeichnis von SELFPHP
:: SELFPHP Forum ::
Fragen rund um die Themen PHP? In über
120.000 Beiträgen finden Sie sicher die passende
Antwort! 
:: Newsletter ::
Abonnieren Sie hier den kostenlosen
SELFPHP Newsletter!
:: Qozido ::
Die Bilderverwaltung mit Logbuch für
Taucher und Schnorchler. 
|
|
PHP5 Klasse für Alexa Web Information Service  |
Beispielaufgabe
Die nachfolgende PHP5 Klasse für Alexa Web Information Service liefert Informationen zu einer bestimmten URL.
Alexa ist ein Dienst, der Daten über Webseitenzugriffe durch Web-Benutzer sammelt und aufbereitet. Auf der Seite von Alexa kann dann zu jeder URL eine Abfrage gemacht werden, um detaillierte Informationen zu einer URL zu erhalten. Vergleichbar mit dem Google PageRank liefert der Alexa Rank die 100.000 meistbesuchten Domains.
Beschreibung
Die Klasse ist so konzipiert, dass die geholten Informationen in einem Array oder als XML aufbereitet und zur Verfügung gestellt werden. Weiterhin besteht die Möglichkeit für jeden Abruf einer Domain beim Alexa Webservice eine XML-Datei zu speichern, die dann mit Datum und Domainnamen gespeichert wird. Diese Datei kann durch die Klasse im nachhinein wieder eingelesen und verwertet werden.
Wie Sie sehen, können Sie mit wenigen Zeilen Programmcode für eine Domain beim Alexa Webservice alle Informationen einholen und als XML-Datei speichern.
Weitere Informationen zum Alexa Webservice finden Sie unter:
http://docs.amazonwebservices.com/AlexaWebInfoService/2005-07-11/
Bitte achten Sie darauf, dass für den Alexa Webservice eine Registrierung notwendig ist. Im Verlauf der Registrierung erhalten Sie dann den öffentlichen und privaten Schlüssel, der für den Webservice von Alexa notwendig ist und den Sie in der Klasse ebenfalls eintragen müssen.
http://aws.amazon.com/awis/
Die Klasse wurde unter der BSD License gestellt und kann somit für private und kommerzielle Zwecke genutzt werden.
<?php
/**
* Alexa_Api_Service (The SELFPHP Alexa_Api_Service PHP5 Class)
*
* PHP5 Class for Alexa Web Information Service
* Required: PHP 5 >= 5.1.2
*
* @package Alexa_Api_Service
* @author Damir Enseleit
* @copyright 2009, SELFPHP OHG
* @license BSD License
* @version 1.0.0
* @link http://www.selfphp.de
*
*/
class Alexa_Api_Service {
/**
* @var string Access Key ID
*/
private $accessKeyID = "";
/**
* @var string Secret Access Key
*/
private $secretAccessKey = "";
/**
* @var string Site URL
*/
private $siteUrl = "";
/**
* @var string Last Error Message
*/
private $lastErrorMessage = "";
/**
* @var string Setzt den Aktion-Parameter.
*
* @link http://docs.amazonwebservices.com/AlexaWebInfoService/2005-07-11/
*/
private $action = "";
/**
* @var string Setzt die Response Group
*
* @link http://docs.amazonwebservices.com/AlexaWebInfoService/2005-07-11/
*/
private $responseGroup = "";
/**
* @var string URL für den Alexa Webservice.
*/
private $serviceUrl = "http://awis.amazonaws.com?";
/**
* @var string Speichert Signatur (Erstellt mit HMAC).
*/
private $storeHmac = "";
/**
* @var string Speichert die Zeit
*/
private $storeTimestamp = "";
/**
* @var string Speichert die abzufragende URL
*/
private $storeServiceUrl = "";
/**
* @var string Speichert den XML-Output vom Webservice
*/
private $storeServiceXmlData = "";
/**
* @var string Speichert den XML-Output vom Webservice
* Folgende Parameter nicht ändern: 'aws:StatusCode', 'Code' oder 'Message' !!!
*
* Der $tagsService arbeitet sehr gut mit ContactInfo z.B.
* $tagsService mit TrafficData ist nicht möglich e.g.
*/
private $tagsService = array('aws:StatusCode','Code','Message');
private $storeServiceArrayData = array();
/**
* Constructor
*
* @param string $accessKey Access Key ID
*
* @param string $secretKey Secret Access Key
*
* @param array $responseArray Ein Array mit den Response Tags
*
*/
function __construct( $accessKey = NULL, $secretKey = NULL, $responseArray = NULL) {
$this->accessKeyID = $accessKey;
$this->secretAccessKey = $secretKey;
$this->setResponseArray( $responseArray );
// Konvertiert alle Tags in Lower Case
for ( $x = 0; $x < count( $this->tagsService ); $x++ ){
$this->tagsService[$x] = strtolower( $this->tagsService[$x] );
}
}
/**
* Definiert die Request Parameter und Response Groups
* @link http://docs.amazonwebservices.com/AlexaWebInfoService/2005-07-11/
*
* @param string $action Setzt den Action Parameter
*
* @param string $responseGroup Setzt die Response Group
*
*/
public function setRequestMode( $action, $responseGroup) {
if ( empty( $action ) || empty( $responseGroup ) ) {
$this->lastErrorMessage = "Error-Message: Request Parameters or Response Groups is empty.";
return false;
}
$this->action = $action;
$this->responseGroup = $responseGroup;
return true;
}
private function setResponseArray( $responseArray ) {
if( count( $responseArray ) > 0 ) {
for ( $x = 0; $x < count( $responseArray ); $x++ ) {
$this->tagsService[] = $responseArray[$x];
}
}
}
/**
* Generiert die Signatur mit HMAC / SHA1
*
* @return bool true / false
*/
private function generateHmac( ) {
if ( empty( $this->action ) || empty( $this->secretAccessKey ) ) {
$this->lastErrorMessage = 'Error-Message: Can\'t generate HMAC - Data or Key is empty.';
return false;
}
$data = $this->action . $this->storeTimestamp;
$this->storeHmac = base64_encode( hash_hmac( 'sha1', $data, $this->secretAccessKey, true ) );
return true;
}
/**
* Zeit erstellen
* Format: yyyy-MM-ddTHH:mm:ss.fffZ
* Z.B. 2009-04-18T11:58:46.000Z
*
* @return bool true
*/
private function generateTimestamp() {
$this->storeTimestamp = gmdate("Y-m-d\\TH:i:s.\\0\\0\\0\\Z", time());
return true;
}
/**
* Baut die URL für die Abfrage auf
*
* @return bool true
*/
private function generateurl() {
$url = 'AWSAccessKeyId=' . $this->accessKeyID
. '&Action=' . $this->action
. '&ResponseGroup=' . $this->responseGroup
. '&Timestamp=' . urlencode( $this->storeTimestamp )
. '&Signature=' . urlencode( $this->storeHmac )
. '&Url=' . urlencode( $this->siteUrl );
$this->storeServiceUrl = $this->serviceUrl . $url;
return true;
}
/**
* Beginnt die Abfrage und speichert das Ergenis als XML
*
* @return bool true
*/
public function beginTransfer() {
unset($this->storeServiceArrayData);
$this->generateTimestamp();
$this->generateHmac();
$this->generateurl();
$ch = curl_init( $this->storeServiceUrl );
curl_setopt( $ch, CURLOPT_TIMEOUT, 5 );
curl_setopt( $ch, CURLOPT_RETURNTRANSFER, 1 );
$result = curl_exec( $ch );
curl_close( $ch );
$this->storeServiceXmlData = $result;
$this->parseXml();
return true;
}
/**
* Liefert den XML-Output vom Webservice
*
* @return string XML-Output
*/
public function getXml() {
return $this->storeServiceXmlData;
}
/**
* Liefert ein Array der abgefragten Daten
*
* @return string XML-Output in einem Array
*/
public function getArray() {
return $this->storeServiceArrayData;
}
/**
* Holt den XML-Output von einer gespeicherten Abfrage aus einer XML-Datei
*
* @param string $path Der Pfad zu der XML-Datei
*
* @return bool true wenn Datei existiert, false falls nicht
*/
public function getStoredXmlData( $path = NULL ) {
if ( file_exists( $path ) ) {
$fp = fopen ( $path, 'r' );
$this->storeServiceXmlData = fread ( $fp, filesize ( $path ) );
fclose ( $fp );
$this->parseXml();
return true;
}
return false;
}
/**
* Speichert den XML-Output in einer Datei
*
* @param string $path Der Pfad zu dem Verzeichnis ohne Slash am Ende
*
* @return string Liefert den kompletten Pfad incl. Dateiname
*/
public function saveXml( $path = 'logs' ) {
if( !is_dir($path)){
mkdir ($path, 0755);
}
$url = ereg_replace( "\\.","-",$this->siteUrl );
$time = gmdate("Y-m-d_H-i-s", time());
$file = $path . '/' . $time . '_' . $url . '.xml';
$fp = fopen ( $file, 'w+' );
fwrite ( $fp, $this->storeServiceXmlData );
fclose ( $fp );
return $file;
}
/**
* Parst die abzurufende URL
*
* @param string $url Die URL
*
* @return bool true / false
*/
public function setUrl( $url = NULL ) {
$errno = "";
$errstr = "";
$url = trim($url);
if (!preg_match("=://=", $url)) {
$url = "http://$url";
}
$url = parse_url($url);
if (!isset($url["port"]))
$url["port"] = 80;
if (!isset($url["path"]))
$url["path"] = "/";
$fp = @fsockopen($url["host"], $url["port"], $errno, $errstr, 30);
if($fp) {
$this->siteUrl = $url["host"];
return true;
}
else {
$this->lastErrorMessage = "Error-Message: $errstr (Error-Number: $errno)";
return false;
}
}
private function tagStart ( $parser, $tagName, $attributes = NULL ) {
$this->tag = $tagName;
}
private function tagEnd ( $parser, $tagName ) {
$this->tag = NULL;
}
private function tagContent ( $parser, $content )
{
$content = trim($content);
if( in_array( strtolower($this->tag), $this->tagsService ) )
$this->storeServiceArrayData[$this->tag] = $content;
}
private function parseXml ()
{
$parser = xml_parser_create();
xml_set_object ( $parser, $this );
xml_set_element_handler ( $parser, 'tagStart', 'tagEnd' );
xml_set_character_data_handler ( $parser, 'tagContent' );
xml_parse ( $parser, $this->storeServiceXmlData );
}
/**
*
*/
function __destruct() {
}
}
?>
|
Anwendungsbeispiel
<?php
include_once ('Alexa_Api_Service.php');
// Access Key - Ersetzen durch eigenen Key
$accessKeyId = 'AccessKey';
// Secret Access Key - Ersetzen durch eigenen Key
$secretAccessKey = 'SecretAccessKey';
// Domain für Abfrage
$site_url = 'selfphp.de';
// Aktion setzen
$action = 'UrlInfo';
// Response Group setzen
// RankByCountry wird nicht unterstützt
$responseGroup = 'Rank,ContactInfo,LinksInCount';
// Response Array setzen
// Flags können aus der XML-Datei genommen werden
$responseArray = array('aws:PhoneNumber','aws:OwnerName','aws:Street','aws:City','aws:Country','aws:Rank');
$alexa = new Alexa_Api_Service( $accessKeyId, $secretAccessKey, $responseArray );
$alexa -> setUrl( $site_url );
$alexa -> setRequestMode ( $action, $responseGroup );
$alexa -> beginTransfer();
// Ergebnis als XML
$getXml = $alexa -> getXml();
echo $getXml;
echo '<br><br>';
// Ergebnis in einem Array
$getArray = $alexa -> getArray();
print_r($getArray);
echo '<br><br>';
// Speichert das XML-Ergebnis in einer Datei.
// Format ist: Date_Time_Domain.xml
// Beispiel: 2009-04-18_16-56-13_selfphp-de.xml
// saveXml('VerzeichnisPfad')
// Der Pfad zum Verzeichnis ohne Slash am Ende.
$the_xml_file_path = $alexa -> saveXml('www-selfphp-de');
echo $the_xml_file_path;
?>
|
Anwendungsbeispiel
<?php
include_once ('Alexa_Api_Service.php');
$responseArray = array('aws:PhoneNumber','aws:OwnerName','aws:Street','aws:City','aws:Country','aws:Rank');
$alexa = new Alexa_Api_Service( NULL, NULL, $responseArray );
$alexa -> getStoredXmlData( "www-selfphp-de/2009-04-27_18-57-03_selfphp-de.xml" );
$xml = $alexa -> getXml();
print_r( $alexa -> getArray() );
?>
|

|
|
|
|
|

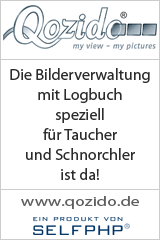
|