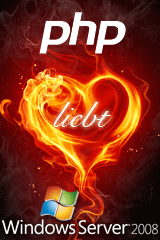
:: Anbieterverzeichnis ::
Globale Branchen
Informieren Sie sich über ausgewählte
Unternehmen im
Anbieterverzeichnis von SELFPHP
:: SELFPHP Forum ::
Fragen rund um die Themen PHP? In über
120.000 Beiträgen finden Sie sicher die passende
Antwort! 
:: Newsletter ::
Abonnieren Sie hier den kostenlosen
SELFPHP Newsletter!
:: Qozido ::
Die Bilderverwaltung mit Logbuch für
Taucher und Schnorchler. 
|
|
Wet Floor Effekt für Vorschaubilder erstellen  |
Beispielaufgabe
Nachfolgend stellen wir Ihnen eine Klasse in PHP5 vor, mit der Sie komfortabel Vorschaubilder erstellen und die generierten Vorschaubilder mit einem Wet Floor Effekt versehen können. Die Klasse ist so konzipiert, dass Sie wahlweise ein oder mehrere Bilder als Vorschaubild mit einem Wet Floor Effekt versehen können. Nachfolgend sehen Sie einige so erstellte Vorschaubilder mit diesem Effekt. Alle angegebenen Bilder werden mit diesem Effekt erstellt und als ein Bild ausgegeben, wahlweise sogar mit Text, Rahmen und Abstand zwischen den Bildern.
Bild 1 - 5 Bilder, weißer Hintergrund, Rahmen 10px, Bildunterschrift, Zellabstand 5 px.
Bild 2 - 4 Bilder, schwarzer Hintergrund, Rahmen 10px, Bildunterschrift, Zellabstand 5 px.
example.php
Nachfolgend sehen Sie den Zugriff auf die Klasse.
<?php
include_once 'wetfloor-effect.php';
$pictures[0] = '5.JPG';
$pictures[1] = '6.JPG';
$pictures[2] = '9.JPG';
$pictures[3] = '7.JPG';
$wetfloor = new WetFloorEffect( );
$wetfloor -> setimages( $pictures );
$wetfloor -> setEnvironment( 10, 5, 600, 180, "000000" );
$wetfloor -> createWetFloor();
$wetfloor -> createText("Copyright 2009 SELFPHP OHG", "4c4c4b", 2);
$wetfloor -> createImage("testbild.jpg", 90);
?>
|
wetfloor-effect.php
Hier sehen Sie noch die vollständige Klasse für die Erzeugung des Vorschaubildes und des Effekts.
<?php
/**
* Create wet floor effect
*
* @author SELFPHP OHG
* @copyright 2009, SELFPHP OHG
* @license BSD License
* @link http://www.selfphp.de
*
*/
class WetFloorEffect {
/**
* @var array Images
*/
private $images = array();
/**
* @var Integer Rahmen um Grafik
*/
private $imgBorder = 0;
/**
* @var Integer Abstand zwischen den einzelnen Bilder
*/
private $imgPadding = 0;
/**
* @var Integer Bildbreite
*/
private $imgWidth = 0;
/**
* @var Integer Bildhöhe
*/
private $imgHeight = 0;
/**
* @var Array Farbe
*/
private $imgRGB = array();
/**
* @var Image Temp Image
*/
private $tempImage = NULL;
/**
* Bilder festlegen
*
* @param array $img Ein Array mit den Pfad zu den Bildern
*
*/
public function setimages( $img = array( NULL ) ){
unset ( $this->images );
for ( $i = 0; $i < count( $img ); $i++ ) {
if ( file_exists( $img[$i] ) )
$this->images[] = $img[$i];
}
}
/**
* Bild-Optionen festlegen
*
* @param integer $border Rahmenbreite
* @param integer $padding Abstand zwischen den Bildern
* @param integer $width Bildbreite
* @param integer $height Bildhöhe
* @param string $backgroundColor Hintergrundfarbe
*
*/
public function setEnvironment( $border = 10, $padding = 5, $width = 200, $height = 100, $backgroundColor = "ffffff"){
$this->imgBorder = $border;
$this->imgPadding = $padding;
$this->imgWidth = $width;
$this->imgHeight = $height;
$this->imgRGB['r'] = hexdec( substr( $backgroundColor, 0, 2 ) );
$this->imgRGB['g'] = hexdec( substr( $backgroundColor, 2, 2 ) );
$this->imgRGB['b'] = hexdec( substr( $backgroundColor, 4, 2 ) );
}
/**
* Effekt erstellen
*
*/
public function createWetFloor(){
$this->tempImage = imagecreatetruecolor( $this->imgWidth, $this->imgHeight );
$color = imagecolorallocate($this->tempImage, $this->imgRGB['r'], $this->imgRGB['g'], $this->imgRGB['b']);
imagefill($this->tempImage, 0, 0, $color);
$picsCount = count( $this->images );
if ( $picsCount == 0 )
return false;
$widthPic = ($this->imgWidth - (( $this->imgBorder * 2) + (( $picsCount - 1) * $this->imgPadding))) / $picsCount;
$heightPic = ($this->imgHeight - (( $this->imgBorder * 2))) - 60;
$left = $this->imgBorder;
for ( $i = 0; $i < $picsCount; $i++ ){
$tempImage = $this->createThumbnail( $this->images[$i], $widthPic, $heightPic);
$tempImage = $this->reflection($tempImage);
$simgx = imagesx($tempImage);
$simgy = imagesy($tempImage);
imagecopyresampled( $this->tempImage, $tempImage, $left, $this->imgBorder, 0, 0, $simgx, $simgy, $simgx, $simgy);
$left += $widthPic + $this->imgPadding;
}
return true;
}
/**
* Spiegelung erstellen
*
*/
private function reflection ( $image ) {
$imageX = imagesx( $image );
$imageY = imagesy( $image );
$gradient = round( $imageY * 0.55 ) - $this->imgBorder;
$imageBack = imagecreatetruecolor( $imageX, $imageY + $gradient );
$color = imagecolorallocate( $imageBack, $this->imgRGB['r'], $this->imgRGB['g'], $this->imgRGB['b'] );
imagefill( $imageBack, 0, 0, $color );
imagecopy( $imageBack, $image, 0, 0, 0, 0, $imageX, $imageY );
$flipImage = imagecreate( $imageX, $imageY );
for ( $y = 0; $y < $imageY; $y++ ) {
imagecopy( $flipImage, $image, 0, $y, 0, $imageY - $y - 1, $imageX, 1 );
}
imagecopy($imageBack, $flipImage, 0, $imageY, 0, 0, $imageX, $imageY);
$alpha = 70 / ( $gradient - 1 );
for ( $i = 0; $i < $gradient; $i++ ) {
if ($i == 0)
$col = imagecolorallocatealpha( $imageBack, $this->imgRGB['r'], $this->imgRGB['g'], $this->imgRGB['b'], 0 );
else
$col = imagecolorallocatealpha( $imageBack, $this->imgRGB['r'], $this->imgRGB['g'], $this->imgRGB['b'], 70 - $i * $alpha );
imageline( $imageBack, 0, $imageY + $i, $imageX, $imageY + $i, $col );
}
return $imageBack;
}
/**
* Text erstellen
*
* @param string $text Der zu zeichnende Text
* @param string $textColor Schriftfarbe
* @param string $size Schriftgröße
*
*/
public function createText( $text = "", $textColor = "ffffff", $size = 4) {
$r = hexdec( substr( $textColor, 0, 2 ) );
$g = hexdec( substr( $textColor, 2, 2 ) );
$b = hexdec( substr( $textColor, 4, 2 ) );
$farbe_b = imagecolorallocate ( $this->tempImage, $r, $g, $b );
imagestring ( $this->tempImage, $size, 10, $this->imgHeight - 20, $text, $farbe_b );
}
/**
* Thumbnail erstellen
*/
private function createThumbnail( $imgSource = NULL, $width, $height ){
$info = getimagesize( $imgSource );
if ( $info[2] == 1 ){
// GIF
$image = imagecreatefromgif( $imgSource );
}
elseif ( $info[2] == 2 ){
// JPG
$image = imagecreatefromjpeg( $imgSource );
}
elseif ( $info[2] == 3 ){
// PNG
$image = imagecreatefrompng( $imgSource );
}
else{
$image = NULL;
}
if ( $image !== Null ){
$imageNew = imagecreatetruecolor( $width, $height );
imagecopyresampled( $imageNew, $image, 0, 0, 0, 0, $width,$height, $info[0], $info[1]);
}
return $imageNew;
}
/**
* Bild speichern
*
* @param string $filedestination Pfad für neue Datei
*
* @param integer $compress Komprimierung
*
*/
public function createImage( $filedestination = NULL, $compress = 85){
imagejpeg($this->tempImage, $filedestination, $compress);
}
public function __deconstruct() {
imagedestroy( $this->tempImage );
}
}
?>
|
Download
WetFloorEffect.zip

|
|
|
|
|

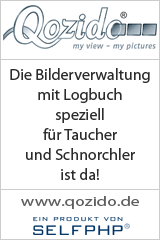
|